Demos
Demo: Accelerometer
This example reads values from Sparkfun's ADXL335 3-axis accelerometer. Because the output of the ADXL335 is between 0-3.3V and because BeagleBone's analogRead pins are 1.8V tolerant, we used a hardware (resistor) voltage divider on each of the accelerometer outputs. This provides a range of 0-1.65V to be read on BeagleBone analogRead pins.
NOTE: Due to output impedance of the ADXL335 being ~32kOhms, a low resistor value for the voltage divider is recommended (between 500 Ohms- 1k Ohms).
We need to do simple math before we continue. Ultimately, we want to know the value in G's being exerted on the accelerometer. The output of the accelerometer is in volts. The first thing we need to do is calculate a formula that will convert volts to Gs for us in our software. This formula for us is:
Example
var b = require('bonescript'); var zeroOffset = 0.4584; var conversionFactor = 0.0917; function callADC(){ b.analogRead('P9_36', printX); b.analogRead('P9_38', printY); b.analogRead('P9_40', printZ); } function printX(x) { var value = (x.value - zeroOffset)/conversionFactor; console.log('Analog Read Value x: ' +value); // when the ADXL335 resting flat on a table or //board, then readings should be x:0 } function printY(x) { var value = (x.value - zeroOffset)/conversionFactor; console.log('Analog Read Value y: ' +value); // when the ADXL335 resting flat on a table or //board, then readings should be y:0 } function printZ(x) { var value = (x.value - zeroOffset)/conversionFactor; console.log('Analog Read Value z: ' +value); // when the ADXL335 resting flat on a table or //board, then readings should be z:1 console.log(''); } //callADC will be invoked 20 times a sec or once every 50 ms var loop = setInterval(callADC, 50); function clear(){ clearInterval(loop); } //after 1 second (1000ms), the interval setTimeout(clear, 1000);
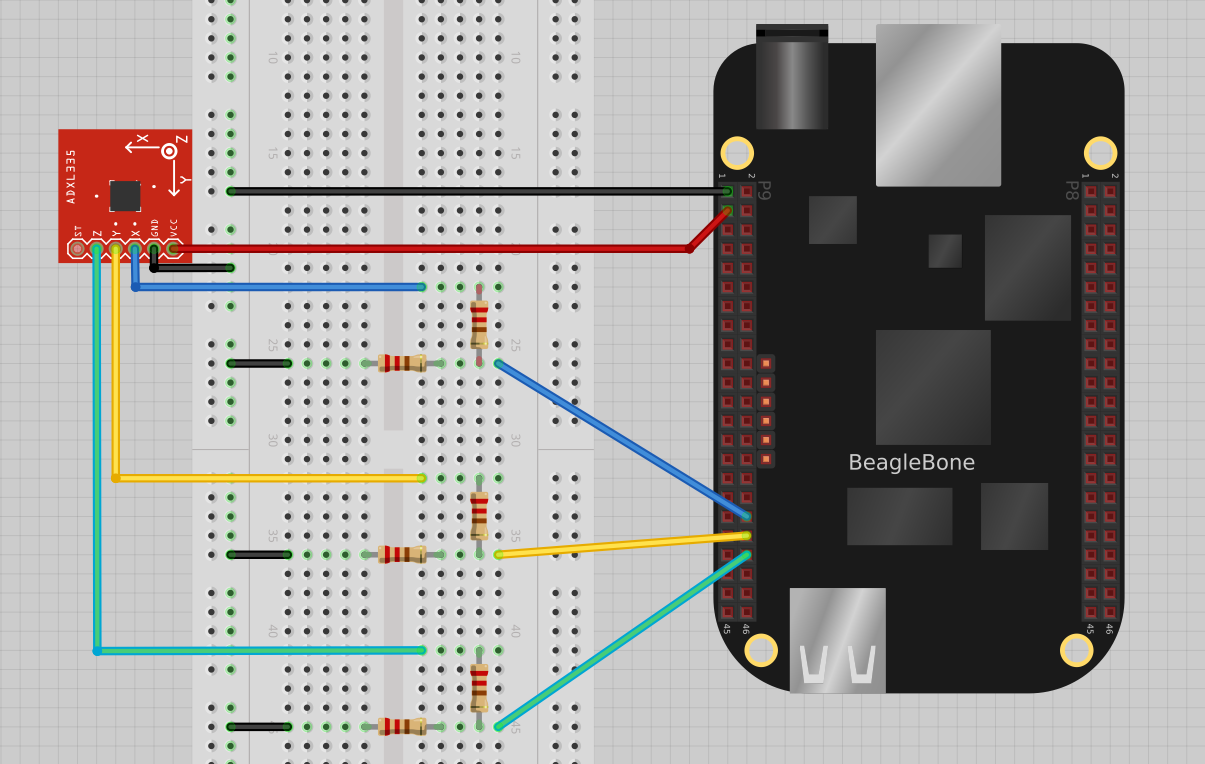
Build and execute instructions
- Hook up BeagleBone to the breadboard as shown in the diagram (above).
- After clicking 'run', notice the console output above for the accelerometer data.
- Experiment by altering the second argument in setTimeout(clear, x) to another number where x is a value in milliseconds (this value determines how long the example will run).